在使用HTTP代理IP实现多线程并发时,主要需要考虑如何管理线程和代理IP的分配。以下是一个基本的实现思路和示例代码,使用Python的threading库和requests库来实现多线程爬虫:
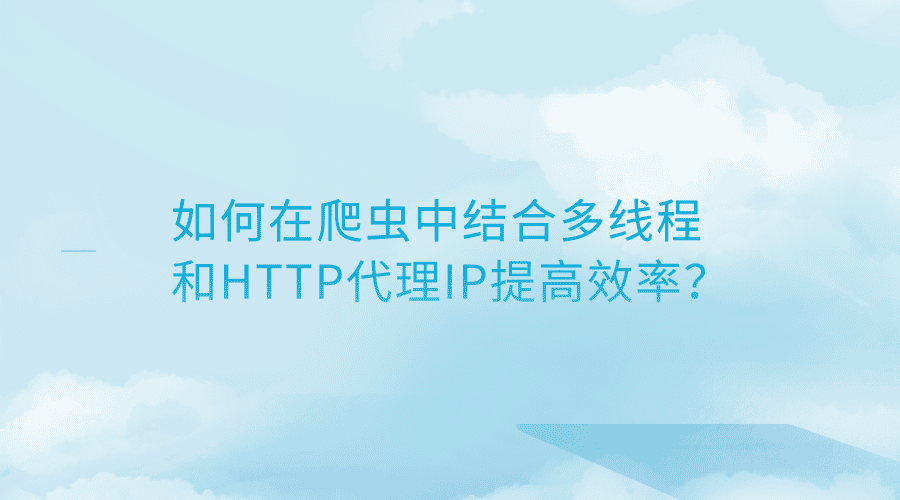
一、实现思路
1、代理池管理
准备一个代理IP池,确保有足够的代理IP供线程使用。
2、线程管理
使用Python的threading库来创建和管理多个线程。
3、请求分发
每个线程从代理池中获取一个代理IP,使用该代理IP发送HTTP请求。
4、异常处理
处理可能出现的网络异常,如连接超时、代理失效等。
二、示例代码
以下是一个简单的示例代码,展示如何使用多线程和代理IP进行并发请求:
import threading
import requests
from queue import Queue
# 代理IP池
proxy_list = [
# 添加更多代理
]
# 任务队列
task_queue = Queue()
# 填充任务队列
urls_to_scrape = [
# 添加更多URL
]
for url in urls_to_scrape:
task_queue.put(url)
# 爬虫线程def worker():
while not task_queue.empty():
url = task_queue.get()
proxy = {'http': proxy_list[task_queue.qsize() % len(proxy_list)]}
try:
response = requests.get(url, proxies=proxy, timeout=5)
print(f"URL: {url}, Status Code: {response.status_code}")
except requests.exceptions.RequestException as e:
print(f"Error fetching {url}: {e}")
finally:
task_queue.task_done()
# 创建线程
num_threads = 5
threads = []
for _ in range(num_threads):
thread = threading.Thread(target=worker)
thread.start()
threads.append(thread)
# 等待所有线程完成
for thread in threads:
thread.join()
三、关键点说明
1、代理池
proxy_list中存储了多个代理IP,供线程使用。
2、任务队列
task_queue用于存储待爬取的URL,确保每个线程都有任务可做。
3、线程函数
worker函数从任务队列中获取URL,并使用代理IP发送请求。
4、异常处理
使用try-except块捕获请求异常,确保程序不会因单个请求失败而中断。
5、线程同步
task_queue.task_done()和thread.join()用于确保所有任务完成后程序才退出。
这种方法可以有效地利用多线程和代理IP进行并发爬取,提高爬虫的效率和稳定性。根据实际需求,可以调整线程数量和代理池大小。